> 구조
- 좌표를 가지는 구조체 백터
- 게임 오브젝트가 구조체 멤버로 가짐
- 캐릭터와 타일은 게임오브젝트를 상속받고 캐릭터는 좌표값을 받아 위치 값을 설정 할 수 있다.
내용 추가
> 맵 좌표
> 튜플 (인덱스 <=> 포지션)
> 매개변수 한정자
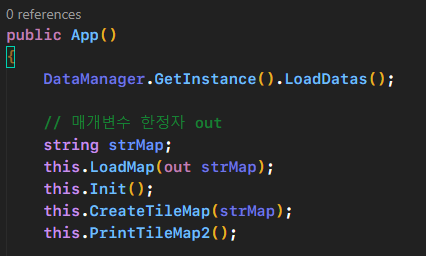
해설 코드
int initializeInMethod;
OutArgExample(out initializeInMethod);
Console.WriteLine(initializeInMethod); // value is now 44
void OutArgExample(out int number)
{
number = 44;
}
※ out 키워드를 사용하면 참조를 통해 인수를 전달할 수 있습니다.
'c# 강의 > 수업내용(문법 관련)' 카테고리의 다른 글
DateTime / Timer 활용 (0) | 2020.04.29 |
---|---|
객체(클래스) JSON 타입으로 입출력 / 폴더생성 (0) | 2020.04.29 |
20200428 - 문자열 콤마 찍기 (0) | 2020.04.28 |
20200427 - 싱글톤 (0) | 2020.04.28 |
20200423 - Achievement (0) | 2020.04.23 |