> 레시피 구매(습득)
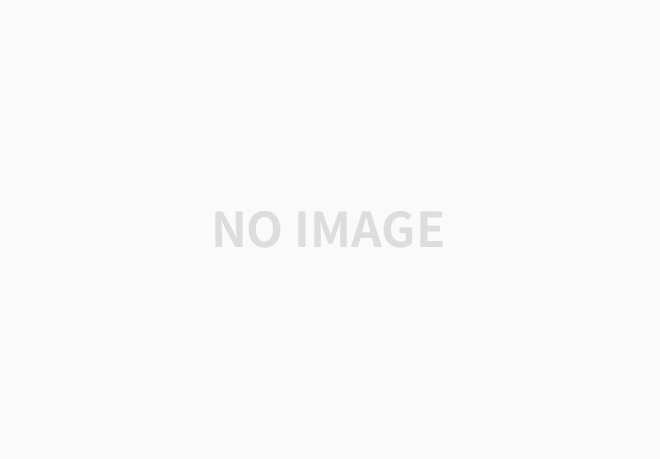
> 구매(습득)한 레시피 확인
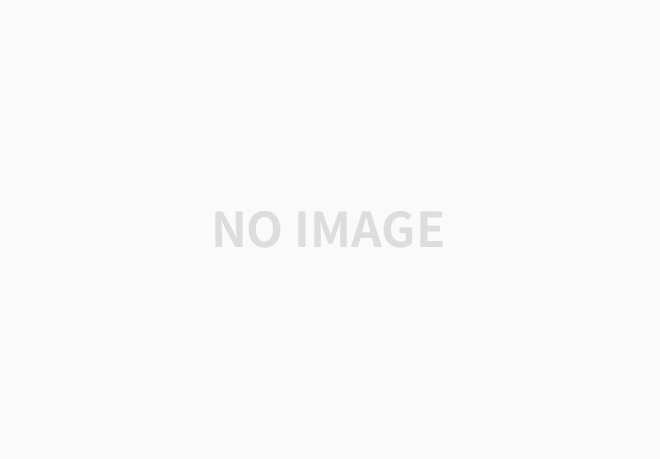
> 레시피의 재료 확인 / 레시피 배우기
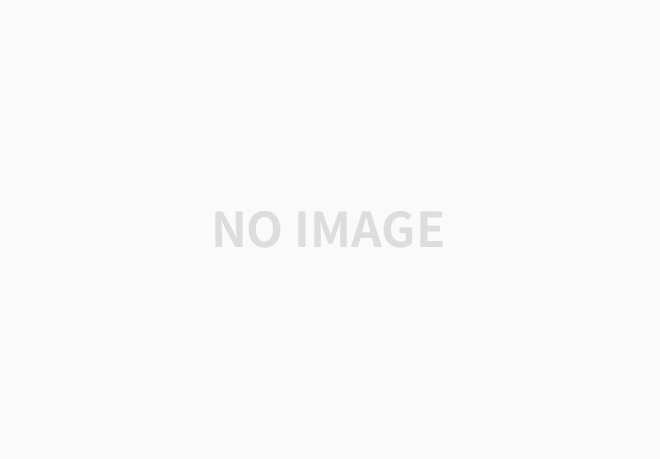
> 배운 레시피 요리 목록
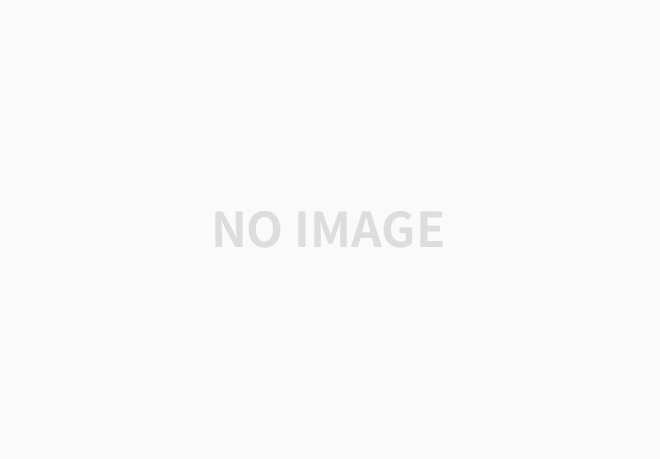
> 요리 하기(가방x - 재료확인 후 요리시작)
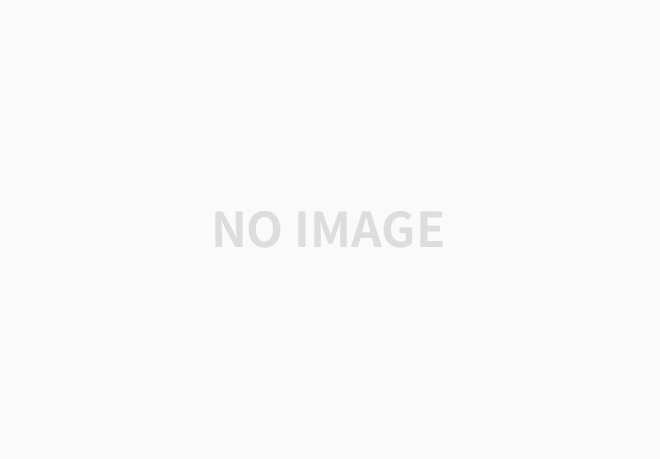
> Food.cs
더보기
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace _20200417_Recipe { class Food { public string name; public Food(string name) { this.name = name; } } }
> Recipe.cs
더보기
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace _20200417_Recipe { class Recipe { public string name; // 레시피 이름(만들어질 음식 이름) public FoodIngredient[] arrFoodIngredientNames; public Recipe(string name, FoodIngredient[] arrFoodIngredientNames) { this.name = name; this.arrFoodIngredientNames = arrFoodIngredientNames; } public string GetName() { return "조리법: " + this.name; } } }
> FoodIngredient
더보기
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace _20200417_Recipe { class FoodIngredient { public string name; public int amount; public FoodIngredient(string name, int amount) { this.name = name; this.amount = amount; } } }
> Character.cs
더보기
using System; using System.Collections.Generic; using System.Data.SqlTypes; using System.Linq; using System.Text; using System.Threading.Tasks; namespace _20200417_Recipe { class Character { public string name; //캐릭터 이름 public Recipe[] arrRecipe; //소지중인 레시피들 public Recipe[] arrCookRecipe; //소지중인 레시피들 public int recipeIndex; // 레시피 배열의 인덱스 public int cookRecipeIndex; // 레시피 배열의 인덱스 public Character(string name) { this.name = name; this.arrRecipe = new Recipe[10]; this.arrCookRecipe = new Recipe[10]; Console.WriteLine($"{this.name}이 생성되었습니다."); } public void PickRecipe(Recipe recipe) { Console.WriteLine("-- 구매 --"); Console.WriteLine($"{recipe.name}"); this.arrRecipe[this.recipeIndex++] = recipe; } public void UseRecipe(string recipe) { Recipe[] newArr = new Recipe[10]; int idx = 0; for (int i = 0; i < this.arrRecipe.Length; i++) { if (this.arrRecipe[i].name == recipe) { this.arrCookRecipe[this.cookRecipeIndex++] = this.arrRecipe[i]; Console.WriteLine(recipe + "레시피를 배웠습니다."); this.arrRecipe[i] = null; foreach (var item in arrRecipe) { if (item != null) { newArr[idx++] = item; } } this.arrRecipe = newArr; this.recipeIndex = -1; break; } } } public Recipe[] PrintMyRecipe() { Console.WriteLine("** 소지하고있는 레시피 **"); foreach (var item in this.arrRecipe) { var idx = Array.IndexOf(this.arrRecipe, item); if (item != null) { Console.WriteLine($"{idx + 1}.{item.name}"); } } return this.arrRecipe; } public Recipe[] PrintCookRecipe() { Console.WriteLine("** 요리가능한 레시피 **"); foreach (var item in this.arrCookRecipe) { var idx = Array.IndexOf(this.arrCookRecipe, item); if (item != null) { Console.WriteLine($"{idx}.{item.name}"); } else { Console.WriteLine("없음"); } } return this.arrCookRecipe; } public Recipe infoRecipe(string recipeName, Recipe[] checkRecipeList) { Recipe recipe = null; foreach (var item in checkRecipeList) { if (item != null) { if (item.name == recipeName) { return item; } } } return recipe; } public string Cook(string recipeName) { // 재료 확인 // 재료 수량 확인 foreach (var item in this.arrCookRecipe) { if (item != null) { if (item.name == recipeName) { Console.Write("-- 재료를 사용합니다: "); foreach (var ingredient in item.arrFoodIngredientNames) { if (ingredient != null) { Console.Write($"[{ingredient.name}({ingredient.amount})] "); } } Console.WriteLine(); } } } Console.WriteLine(recipeName + "를 요리합니다."); return ""; } } }
> App.cs
더보기
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace _20200417_Recipe { class App { Character hong; FoodIngredient[] arrFoodIngredientNames; Recipe recipe; string input = ""; bool buyed1 = false; bool buyed2 = false; public App() { this.hong = new Character("홍길동"); while (true) { MainView(); Console.Write(">입력: "); input = Console.ReadLine(); SubView(input); } } public void MainView() { Console.WriteLine(); Console.WriteLine("■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■"); Console.WriteLine("[1.요리하기] [2.레시피 구매] [3.가방 레시피 확인]"); Console.WriteLine("■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■■"); } public void SubView(string inputNum) { switch (inputNum) { case "1": Recipe[] result = this.hong.PrintCookRecipe(); CookRecipe(result); break; case "2": BuyRecipe(); break; case "3": Recipe[] arrRecipeList = this.hong.PrintMyRecipe(); Console.WriteLine(); Console.WriteLine("> [1.레시피 재료확인] [2.요리배우기] [9.돌아가기]"); Console.Write("> 입력: "); input = Console.ReadLine(); if (input == "9") { Console.WriteLine(">이전으로 돌아갑니다!"); return; } if (input == "1") { Console.Write("> 확인하려는 요리 번호를 입력해주세요: "); input = Console.ReadLine(); infoRecipe(input, arrRecipeList); } Console.WriteLine(); Console.WriteLine("> 배우시려면 해당 번호 입력, [9.돌아가기]"); Console.Write(">입력: "); input = Console.ReadLine(); if (input == "9") { Console.WriteLine(">이전으로 돌아갑니다!"); return; } UseRecipe(input, arrRecipeList); break; default: break; } } public void infoRecipe(string input, Recipe[] checkRecipeList) { int check = int.Parse(input); Recipe result = hong.infoRecipe(checkRecipeList[check - 1].name, checkRecipeList); if (result != null) { Console.WriteLine("> 찾은 요리정보 <"); Console.Write($"{result.name} : 필수재료--"); foreach (var item in result.arrFoodIngredientNames) { Console.Write($"[{item.name}({item.amount})] "); } } else { Console.WriteLine("찾는 요리 없음"); } Console.WriteLine(); } public void CookRecipe(Recipe[] arrCookRecipe) { Console.WriteLine("ㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡㅡ"); Console.WriteLine("> 요리시려면 해당 번호 입력, [9.돌아가기]"); Console.Write("> 입력: "); input = Console.ReadLine(); if (input == "9") { Console.WriteLine(">이전으로 돌아갑니다!"); return; } switch (input) { case "0": if (arrCookRecipe[0] == null) { return; } this.hong.Cook(arrCookRecipe[0].name); break; case "1": if (arrCookRecipe[1] == null) { return; } this.hong.Cook(arrCookRecipe[1].name); break; default: Console.WriteLine("> 없는번호입니다."); break; } Console.WriteLine(); } public void UseRecipe(string input, Recipe[] arrRecipeList) { switch (input) { case "1": if (arrRecipeList[0] == null) { return; } this.hong.UseRecipe(arrRecipeList[0].name); break; case "2": if (arrRecipeList[1] == null) { return; } this.hong.UseRecipe(arrRecipeList[1].name); break; default: Console.WriteLine("> 없는번호입니다."); break; } Console.WriteLine(); } public void BuyRecipe() { FoodIngredient foodIngredient1; FoodIngredient foodIngredient2; Console.WriteLine("> [1.햄버거] [2.괴물 오믈렛]"); Console.Write(">입력: "); input = Console.ReadLine(); switch (input) { case "1": if (!buyed1) { // 기본 레시피1 생성 foodIngredient1 = new FoodIngredient("쇠고기 패티", 1); foodIngredient2 = new FoodIngredient("빵", 1); this.arrFoodIngredientNames = new FoodIngredient[2]; this.arrFoodIngredientNames[0] = foodIngredient1; this.arrFoodIngredientNames[1] = foodIngredient2; this.recipe = new Recipe("햄버거", this.arrFoodIngredientNames); this.hong.PickRecipe(this.recipe); this.buyed1 = true; } else { Console.WriteLine("> 해당 레시피는 더이상 구매 불가"); } break; case "2": if (!buyed2) { // 기본 레시피2 생성 foodIngredient1 = new FoodIngredient("거대한 알", 1); foodIngredient2 = new FoodIngredient("독특한 양념", 1); this.arrFoodIngredientNames = new FoodIngredient[2]; this.arrFoodIngredientNames[0] = foodIngredient1; this.arrFoodIngredientNames[1] = foodIngredient2; this.recipe = new Recipe("괴물 오믈렛", this.arrFoodIngredientNames); this.hong.PickRecipe(this.recipe); this.buyed2 = true; } else { Console.WriteLine("> 해당 레시피는 더이상 구매 불가"); } break; default: break; } Console.WriteLine(); } } //class end }
'c# 강의 > 과제' 카테고리의 다른 글
20200421 - File 읽기 (JSON) (0) | 2020.04.22 |
---|---|
20200420 - List 과제 (0) | 2020.04.20 |
20200415 - 아이템 생성, 검색, 꺼내기, 출력 과제 (0) | 2020.04.16 |
20200414 - 테란~아카데미까지 (0) | 2020.04.14 |
20200413 - 슈팅 게임 (0) | 2020.04.13 |